Tag Archives: .NET

Implement a secure Blazor Web application using OpenID Connect and security headers
This article shows how to implement a secure .NET 8 Blazor Web application using OpenID Connect and security headers with CSP nonces. The NetEscapades.AspNetCore.SecurityHeaders nuget package is used to implement the security headers and OpenIddict is used to implement the OIDC server. Code: https://github.com/damienbod/BlazorWebOidc OpenIddict is used as the identity provider and an OpenID connect […]

BFF secured ASP.NET Core application using downstream API and an OAuth client credentials JWT
This article shows how to implement a web application using backend for frontend security architecture for authentication and consumes data from a downstream API protected using a JWT access token which can only be accessed using an app-to-app access token. The access token is acquired using the OAuth2 client credentials flow and the API does […]
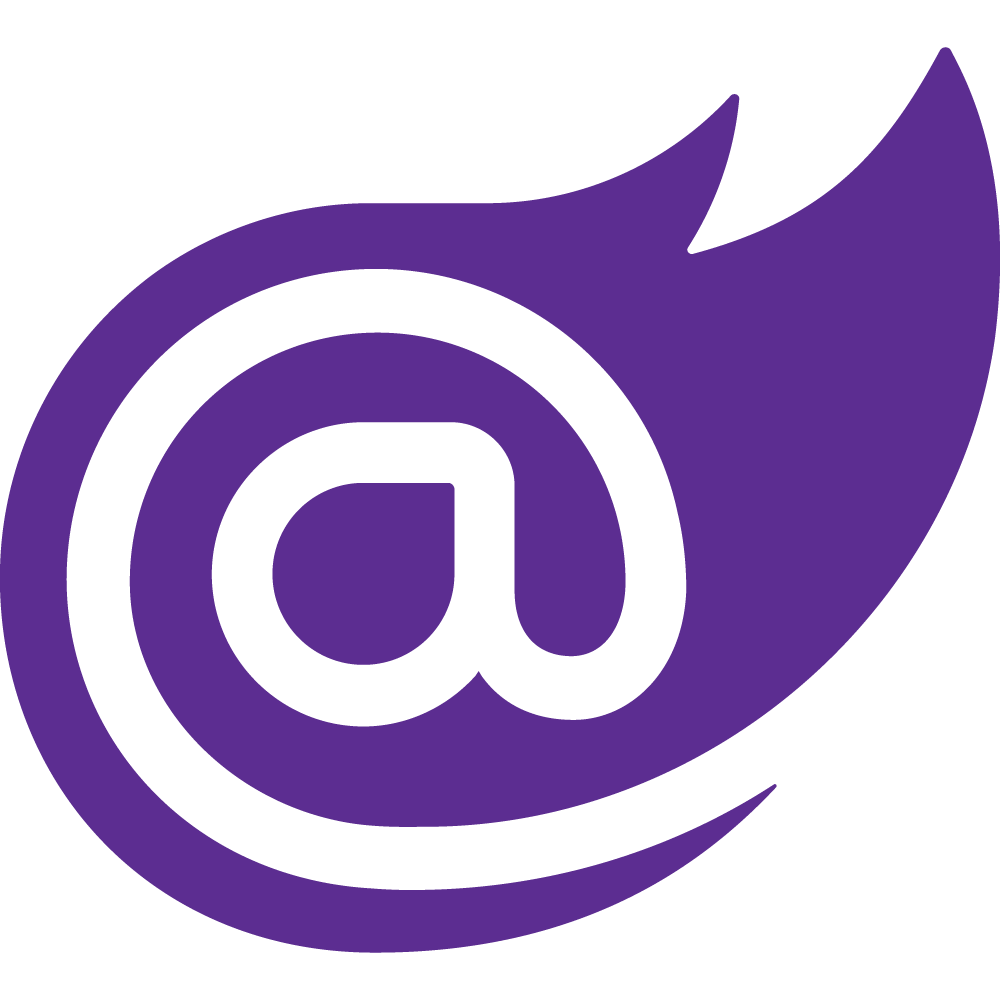
Using a CSP nonce in Blazor Web
This article shows how to use a CSP nonce in a Blazor Web application using the InteractiveServer server render mode. Using a CSP nonce is a great way to protect web applications against XSS attacks and other such Javascript vulnerabilities. Code: https://github.com/damienbod/BlazorServerOidc Notes The code in this example was built using the example provided by […]
Basic Authentication in ASP.NET Core
This article shows how basic authentication could be implemented in an ASP.NET Core application. This is not the recommended way to implement security for user flows as the password is always sent with each request but the flow is sometimes required to implement a standard or you sometimes need to support one side of an […]

Force MFA in Blazor using Azure AD and Continuous Access
This article shows how to force MFA from your application using Azure AD and a continuous access auth context. When producing software which can be deployed to multiple tenants, instead of hoping IT admins configure this correctly in their tenants, you can now force this from the application. Many tenants do not force MFA. Code: […]

Implement Azure AD Continuous Access in an ASP.NET Core Razor Page app using a Web API
This article shows how Azure AD continuous access (CA) can be used in an ASP.NET Core UI application to force MFA when using an administrator API from a separate ASP.NET Core application. Both applications are secured using Microsoft.Identity.Web. An ASP.NET Core Razor Page application is used to implement the UI application. The API is implemented […]

Securing multiple Auth0 APIs in ASP.NET Core using OAuth Bearer tokens
This article shows a strategy for security multiple APIs which have different authorization requirements but the tokens are issued by the same authority. Auth0 is used as the identity provider. A user API and a service API are implemented in the ASP.NET Core API project. The access token for the user API data is created […]
Search Queries and Filters with ElasticsearchCRUD
This article demonstrates how to do searches with ElasticsearchCRUD. The API provides a search model which can be used with the search API from Elasticsearch. You can search both sync/async and also provide you own JSON string or use the search model. Lots of examples can be found here: https://github.com/damienbod/ElasticsearchCRUD/tree/master/src/ElasticsearchCRUDNUnit.Integration.Test Other Tutorials: Part 1: ElasticsearchCRUD […]
Elasticsearch CRUD .NET provider
The article explains how to use the ElasticsearchCRUD NuGet package. ElasticsearchCRUD is designed so that you can do CRUD operations for any entity and insert, delete, update or select single documents from Elasticsearch. The package only includes basic search or query possibilities. Code: https://github.com/damienbod/ElasticsearchCRUD NuGet Package: https://www.nuget.org/packages/ElasticsearchCRUD/ Issues: https://github.com/damienbod/ElasticsearchCRUD/issues Tutorials: Part 1: ElasticsearchCRUD introduction Part […]