This article shows how to implement the OpenID Connect Code Flow with PKCE using OpenIddict hosted in an ASP.NET Core application, an ASP.NET Core Web API and an Angular application as the client.
Code: https://github.com/damienbod/AspNetCoreOpeniddict
2021-12-24 Updated to ASP.NET Core 6, Angular 13
2020-12-26 Updated to ASP.NET Core 5, Angular 11, OpenIddict 3
2017-05-27 Updated to ASP.NET Core 2.1, Angular 6.0.3
2017-11-24 Updated to ASP.NET Core 2, Angular 5, angular-auth-oidc-client
Three different projects are used to implement the application. The OpenIddict Server with adds the Code Flow with PKCE is used to authenticate and authorise, the resource server is used to provide the API and uses introspection to validate the HTTP requests and the access tokens and the Angular application implements the UI.
OpenIddict Server implementing the Code Flow with PKCE
NuGet package manager is used to download the required packages. You need to download and install the correct OpenIddict packages for the token server.

Or you can just add them directly to the csproj file.
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net6.0</TargetFramework>
</PropertyGroup>
<ItemGroup>
<None Remove="usersdatabase.sqlite" />
</ItemGroup>
<ItemGroup>
<Content Include="usersdatabase.sqlite">
<CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory>
</Content>
</ItemGroup>
<ItemGroup>
<PackageReference Include="Microsoft.AspNetCore.Identity.EntityFrameworkCore" Version="6.0.1" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Design" Version="6.0.1">
<PrivateAssets>all</PrivateAssets>
<IncludeAssets>runtime; build; native; contentfiles; analyzers; buildtransitive</IncludeAssets>
</PackageReference>
<PackageReference Include="Microsoft.EntityFrameworkCore.Sqlite" Version="6.0.1" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Tools" Version="6.0.1">
<PrivateAssets>all</PrivateAssets>
<IncludeAssets>runtime; build; native; contentfiles; analyzers; buildtransitive</IncludeAssets>
</PackageReference>
<PackageReference Include="OpenIddict" Version="3.1.1" />
<PackageReference Include="OpenIddict.AspNetCore" Version="3.1.1" />
<PackageReference Include="OpenIddict.EntityFrameworkCore" Version="3.1.1" />
<PackageReference Include="OpenIddict.Quartz" Version="3.1.1" />
<PackageReference Include="OpenIddict.Server.AspNetCore" Version="3.1.1" />
<PackageReference Include="Quartz.Extensions.Hosting" Version="3.3.3" />
<PackageReference Include="BuildBundlerMinifier" Version="3.2.449" />
<PackageReference Include="Serilog.Settings.Configuration" Version="3.1.0" />
<PackageReference Include="Serilog" Version="2.10.0" />
<PackageReference Include="Serilog.AspNetCore" Version="4.1.0" />
<PackageReference Include="Serilog.Settings.Configuration" Version="3.3.0" />
<PackageReference Include="Serilog.Sinks.Console" Version="4.0.1" />
<PackageReference Include="Serilog.Sinks.File" Version="5.0.0" />
</ItemGroup>
<Target Name="DebugEnsureNodeEnv" BeforeTargets="Build" Condition=" '$(Configuration)' == 'Debug' And !Exists('node_modules') ">
<!-- Ensure Node.js is installed -->
<Exec Command="node --version" ContinueOnError="true">
<Output TaskParameter="ExitCode" PropertyName="ErrorCode" />
</Exec>
<Error Condition="'$(ErrorCode)' != '0'" Text="Node.js is required to build and run this project. To continue, please install Node.js from https://nodejs.org/, and then restart your command prompt or IDE." />
<Message Importance="high" Text="Restoring dependencies using 'npm'. This may take several minutes..." />
<Exec WorkingDirectory="" Command="npm install" />
</Target>
</Project>
The OpenIddict packages are configured in the ConfigureServices and the Configure methods in the Startup class. The following code configures the OpenID Connect server with a SQLite database using Entity Framework Core. The required endpoints are enabled, and Json Web tokens are used.
public void ConfigureServices(IServiceCollection services)
{
services.AddControllersWithViews();
services.AddDbContext<ApplicationDbContext>(options =>
{
// Configure the context to use Microsoft SQL Server.
options.UseSqlite(Configuration.GetConnectionString("DefaultConnection"));
// Register the entity sets needed by OpenIddict.
// Note: use the generic overload if you need
// to replace the default OpenIddict entities.
options.UseOpenIddict();
});
// Register the Identity services.
services.AddIdentity<ApplicationUser, IdentityRole>()
.AddEntityFrameworkStores<ApplicationDbContext>()
.AddDefaultTokenProviders();
services.AddCors(options =>
{
options.AddPolicy("AllowAllOrigins",
builder =>
{
builder
.AllowCredentials()
.WithOrigins(
"https://localhost:4200")
.SetIsOriginAllowedToAllowWildcardSubdomains()
.AllowAnyHeader()
.AllowAnyMethod();
});
});
// Configure Identity to use the same JWT claims as OpenIddict instead
// of the legacy WS-Federation claims it uses by default (ClaimTypes),
// which saves you from doing the mapping in your authorization controller.
services.Configure<IdentityOptions>(options =>
{
options.ClaimsIdentity.UserNameClaimType = Claims.Name;
options.ClaimsIdentity.UserIdClaimType = Claims.Subject;
options.ClaimsIdentity.RoleClaimType = Claims.Role;
});
// OpenIddict offers native integration with Quartz.NET to perform scheduled tasks
// (like pruning orphaned authorizations/tokens from the database) at regular intervals.
services.AddQuartz(options =>
{
options.UseMicrosoftDependencyInjectionJobFactory();
options.UseSimpleTypeLoader();
options.UseInMemoryStore();
});
// Register the Quartz.NET service and configure it to block shutdown until jobs are complete.
services.AddQuartzHostedService(options => options.WaitForJobsToComplete = true);
services.AddOpenIddict()
// Register the OpenIddict core components.
.AddCore(options =>
{
// Configure OpenIddict to use the Entity Framework Core stores and models.
// Note: call ReplaceDefaultEntities() to replace the default OpenIddict entities.
options.UseEntityFrameworkCore()
.UseDbContext<ApplicationDbContext>();
// Developers who prefer using MongoDB can remove the previous lines
// and configure OpenIddict to use the specified MongoDB database:
// options.UseMongoDb()
// .UseDatabase(new MongoClient().GetDatabase("openiddict"));
// Enable Quartz.NET integration.
options.UseQuartz();
})
// Register the OpenIddict server components.
.AddServer(options =>
{
// Enable the authorization, device, logout, token, userinfo and verification endpoints.
options.SetAuthorizationEndpointUris("/connect/authorize")
.SetDeviceEndpointUris("/connect/device")
.SetLogoutEndpointUris("/connect/logout")
.SetIntrospectionEndpointUris("/connect/introspect")
.SetTokenEndpointUris("/connect/token")
.SetUserinfoEndpointUris("/connect/userinfo")
.SetVerificationEndpointUris("/connect/verify");
// Note: this sample uses the code, device code, password and refresh token flows, but you
// can enable the other flows if you need to support implicit or client credentials.
options.AllowAuthorizationCodeFlow()
.AllowDeviceCodeFlow()
.AllowHybridFlow()
.AllowRefreshTokenFlow();
// Mark the "email", "profile", "roles" and "dataEventRecords" scopes as supported scopes.
options.RegisterScopes(Scopes.Email, Scopes.Profile, Scopes.Roles, "dataEventRecords");
// Register the signing and encryption credentials.
options.AddDevelopmentEncryptionCertificate()
.AddDevelopmentSigningCertificate();
// Force client applications to use Proof Key for Code Exchange (PKCE).
options.RequireProofKeyForCodeExchange();
// Register the ASP.NET Core host and configure the ASP.NET Core-specific options.
options.UseAspNetCore()
.EnableStatusCodePagesIntegration()
.EnableAuthorizationEndpointPassthrough()
.EnableLogoutEndpointPassthrough()
.EnableTokenEndpointPassthrough()
.EnableUserinfoEndpointPassthrough()
.EnableVerificationEndpointPassthrough()
.DisableTransportSecurityRequirement(); // During development, you can disable the HTTPS requirement.
// Note: if you don't want to specify a client_id when sending
// a token or revocation request, uncomment the following line:
//
// options.AcceptAnonymousClients();
// Note: if you want to process authorization and token requests
// that specify non-registered scopes, uncomment the following line:
//
// options.DisableScopeValidation();
// Note: if you don't want to use permissions, you can disable
// permission enforcement by uncommenting the following lines:
//
// options.IgnoreEndpointPermissions()
// .IgnoreGrantTypePermissions()
// .IgnoreResponseTypePermissions()
// .IgnoreScopePermissions();
// Note: when issuing access tokens used by third-party APIs
// you don't own, you can disable access token encryption:
//
// options.DisableAccessTokenEncryption();
})
// Register the OpenIddict validation components.
.AddValidation(options =>
{
// Configure the audience accepted by this resource server.
// The value MUST match the audience associated with the
// "demo_api" scope, which is used by ResourceController.
options.AddAudiences("rs_dataEventRecordsApi");
// Import the configuration from the local OpenIddict server instance.
options.UseLocalServer();
// Register the ASP.NET Core host.
options.UseAspNetCore();
// For applications that need immediate access token or authorization
// revocation, the database entry of the received tokens and their
// associated authorizations can be validated for each API call.
// Enabling these options may have a negative impact on performance.
//
// options.EnableAuthorizationEntryValidation();
// options.EnableTokenEntryValidation();
});
services.AddTransient<IEmailSender, AuthMessageSender>();
services.AddTransient<ISmsSender, AuthMessageSender>();
// Register the worker responsible of seeding the database with the sample clients.
// Note: in a real world application, this step should be part of a setup script.
services.AddHostedService<Worker>();
}
The Configure method and the authentication middleware.
public void Configure(IApplicationBuilder app)
{
app.UseDeveloperExceptionPage();
app.UseCors("AllowAllOrigins");
app.UseStaticFiles();
app.UseStatusCodePagesWithReExecute("/error");
app.UseRouting();
app.UseRequestLocalization(options =>
{
options.AddSupportedCultures("en-US", "fr-FR");
options.AddSupportedUICultures("en-US", "fr-FR");
options.SetDefaultCulture("en-US");
});
app.UseAuthentication();
app.UseAuthorization();
app.UseEndpoints(options => options.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}"));
}
Entity Framework Core database migrations:
dotnet ef migrations add test
dotnet ef database update test
The UserinfoController controller is used to return user data to the client. The API requires a token which is validated using the JWT Bearer token validation, configured in the Startup class.
The required claims need to be added here, as the application requires. This example adds some extra role claims which are used in the Angular SPA.
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Authentication;
using Microsoft.AspNetCore.Authorization;
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Mvc;
using OpeniddictServer.Models;
using OpenIddict.Abstractions;
using OpenIddict.Server.AspNetCore;
using static OpenIddict.Abstractions.OpenIddictConstants;
namespace OpeniddictServer.Controllers
{
public class UserinfoController : Controller
{
private readonly UserManager<ApplicationUser> _userManager;
public UserinfoController(UserManager<ApplicationUser> userManager)
=> _userManager = userManager;
[Authorize(AuthenticationSchemes = OpenIddictServerAspNetCoreDefaults.AuthenticationScheme)]
[HttpGet("~/connect/userinfo"), HttpPost("~/connect/userinfo")]
[IgnoreAntiforgeryToken, Produces("application/json")]
public async Task<IActionResult> Userinfo()
{
var user = await _userManager.GetUserAsync(User);
if (user is null)
{
return Challenge(
authenticationSchemes: OpenIddictServerAspNetCoreDefaults.AuthenticationScheme,
properties: new AuthenticationProperties(new Dictionary<string, string>
{
[OpenIddictServerAspNetCoreConstants.Properties.Error] = Errors.InvalidToken,
[OpenIddictServerAspNetCoreConstants.Properties.ErrorDescription] =
"The specified access token is bound to an account that no longer exists."
}));
}
var claims = new Dictionary<string, object>(StringComparer.Ordinal)
{
// Note: the "sub" claim is a mandatory claim and must be included in the JSON response.
[Claims.Subject] = await _userManager.GetUserIdAsync(user)
};
if (User.HasScope(Scopes.Email))
{
claims[Claims.Email] = await _userManager.GetEmailAsync(user);
claims[Claims.EmailVerified] = await _userManager.IsEmailConfirmedAsync(user);
}
if (User.HasScope(Scopes.Phone))
{
claims[Claims.PhoneNumber] = await _userManager.GetPhoneNumberAsync(user);
claims[Claims.PhoneNumberVerified] = await _userManager.IsPhoneNumberConfirmedAsync(user);
}
if (User.HasScope(Scopes.Roles))
{
claims[Claims.Role] = await _userManager.GetRolesAsync(user);
}
// Note: the complete list of standard claims supported by the OpenID Connect specification
// can be found here: http://openid.net/specs/openid-connect-core-1_0.html#StandardClaims
return Ok(claims);
}
}
}
The AuthorizationController controller implements the Authorize method where the claims can be added to the tokens as required. The flow in this example requires the authorization code flow..
If you require more examples, or different flows, refer to the excellent openiddict-samples .
Angular Code Flow with PKCE client
The Angular application uses the AuthConfiguration class to set the options required for the OpenID Connect Code Flow. The ‘code’ is defined as the response type so that an access_token is returned as well as the id_token using the OIDC code flow with a PKCE. The jwks_url is required so that the client can ge the signiture from the server to validate the token. The userinfo_url and the logoutEndSession_url are used to define the user data url and the logout url. The configuration here has to match the configuration on the server.
The OidcSecurityService is used to send the login request to the server and also handle the callback which validates the tokens. This class also persists the token data to the local storage.
The OidcSecurityValidation class defines the functions used to validate the tokens defined in the OpenID Connect specification for the Code Flow with PKCE.
jsrsasign is used to validate the token signature.
Once logged into the application, the access_token is added to the header of each request and sent to the resource server or the required APIs on the OpenIddict server.
private setHeaders(): any {
this.headers = new HttpHeaders();
this.headers = this.headers.set('Content-Type', 'application/json');
this.headers = this.headers.set('Accept', 'application/json');
const token = this.securityService.getAccessToken();
if (token !== '') {
const tokenValue = 'Bearer ' + token;
this.headers = this.headers.append('Authorization', tokenValue);
}
}
ASP.NET Core Resource Server API
The resource server provides an API protected by authorization policies
using ResourceServer.Model;
using ResourceServer.Repositories;
using Microsoft.AspNetCore.Authorization;
using Microsoft.AspNetCore.Mvc;
namespace ResourceServer.Controllers
{
[Authorize("dataEventRecordsPolicy")]
[Route("api/[controller]")]
public class DataEventRecordsController : Controller
{
private readonly DataEventRecordRepository _dataEventRecordRepository;
public DataEventRecordsController(DataEventRecordRepository dataEventRecordRepository)
{
_dataEventRecordRepository = dataEventRecordRepository;
}
[HttpGet]
public IActionResult Get()
{
return Ok(_dataEventRecordRepository.GetAll());
}
[HttpGet("{id}")]
public IActionResult Get(long id)
{
return Ok(_dataEventRecordRepository.Get(id));
}
[HttpPost]
public void Post([FromBody]DataEventRecord value)
{
_dataEventRecordRepository.Post(value);
}
[HttpPut("{id}")]
public void Put(long id, [FromBody]DataEventRecord value)
{
_dataEventRecordRepository.Put(id, value);
}
[HttpDelete("{id}")]
public void Delete(long id)
{
_dataEventRecordRepository.Delete(id);
}
}
}
The policies are implemented in the Startup class and the scope dataEventRecords.
services.AddScoped<IAuthorizationHandler, RequireScopeHandler>();
services.AddAuthorization(options =>
{
options.AddPolicy("dataEventRecordsPolicy", policyUser =>
{
policyUser.Requirements.Add(new RequireScope());
});
});
Introspection is used to validate the API HTTP requests.
services.AddAuthentication(options =>
{
options.DefaultScheme = OpenIddictValidationAspNetCoreDefaults.AuthenticationScheme;
});
// Register the OpenIddict validation components.
services.AddOpenIddict()
.AddValidation(options =>
{
// Note: the validation handler uses OpenID Connect discovery
// to retrieve the address of the introspection endpoint.
options.SetIssuer("https://localhost:44395/");
options.AddAudiences("rs_dataEventRecordsApi");
// Configure the validation handler to use introspection and register the client
// credentials used when communicating with the remote introspection endpoint.
options.UseIntrospection()
.SetClientId("rs_dataEventRecordsApi")
.SetClientSecret("dataEventRecordsSecret");
// Register the System.Net.Http integration.
options.UseSystemNetHttp();
// Register the ASP.NET Core host.
options.UseAspNetCore();
});
Running the application
When the application is started, all 3 applications are run, using the Visual Studio multiple project start option.
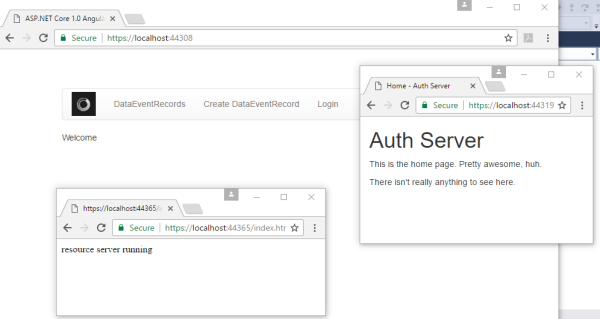
After the user clicks the login button, the user is redirected to the OpenIddict server to login.

After a successful login, the user is redirected back to the Angular application.

Links:
https://github.com/openiddict/openiddict-core
https://github.com/robinvanderknaap/authorization-server-openiddict
https://github.com/openiddict/openiddict-core/issues/49
https://github.com/openiddict/openiddict-samples
https://www.scottbrady91.com/OpenID-Connect/OpenID-Connect-Flows
Hi,
I have implemented your code, but while testing am getting the following error in my angular app.
Unexpected token ( in JSON at position 1.
This error comes when AuthorizeCallback tries to decode the base64 for the token.
Thanks,
Hi I have done a large update, you need to build the client app as well.
npm install
npm run build-production
Greetings Damien
I’m getting the same error when trying to use the latest version. It looks like it’s trying to parse the id_token as json, but the decoded version is not json.
Extremely helpful, thank you!
I’m adapting to use a SQL db, (I use db first so I’m working with the sql tables). I’ve gotten the client_id and redirect uri’s correct, but am at the stage when I click “login” I’m getting:
error:invalid_request
error_description:Confidential clients are not allowed to retrieve an access token from the authorization endpoint.
Can you point me in the right direction?
Todd
Got it, it was the “Type” field of table OpenIddictApplications (was set to confidential, changed to public).
Reblogged this on sharepointswe.
All I get is “This is server routing, not angular routing”
Hi Alfetta159, In the startup class you need to add the angular route so it won’t be handled by the server routing.
Greetings Damien
What is the point of not having it added?
And how do I add it?
You might want to use server routing for files, or MVC or whatever
I’ve just updated code to OpenIddict 2.0.0-rc2-0792 and have successfully integrated custom OpenIddict table names using db first dotnet ef scaffolding. Server app coming up fine, angular app returning as above (“This is server routing…”). I’m sure this reflects my lack of familiarity of working with an angular app in a Visual Studio 2017 solution/project. Anything you can add to clarify the solution would be greatly appreciated
In the startup class you need to add all the client routes, so that the MVC server app does n0t try to route it.
[…] There are some good examples of how to do this on the web, such as this article by Kévin Chalet or this one by Damien Bowden. Additionally, there are the ASP.NET Core/JavaScript samples for OpenIddict […]
Great article.
Can this code be updated to Core 2.0 and Angular 5 with latest openiddict please?
yes, as soon as possible
Many Thanks
Will wait for it.
Any plans soon to migrate to Core 2 and Angular 5?
Updated ASP.NET Core 2, Angular 5, angular-auth-oidc-client
https://github.com/damienbod/AspNetCoreOpeniddictAngularImplicitFlow
Thanks a lot. This is exactly what was required. I have been a keen follower of your blogs.
I tested the latest code.
The authentication part is working OK.
But while accessing resource server, it is throwing 404 error.
Any help?
The latest update works perfectly.
Thanks a lot.
A great article.
I pulled your latest rep from github, and when I startup the project in vs2017, I an error “This site can’t be reached
The webpage at https://localhost:44319/ might be temporarily down or it may have moved permanently to a new web address.”
hi Clint, you might need to do a npm install, then a npm run build-dev, greetings Damien
I have all the components running – auth server, resource server and the client. I was able to register a user via the UI, I can see the user in the DB. However when I try to log in (with correct credentials, I’m sure of it), I get a 400 Bad Request response from the auth server with the following message:
error:invalid_request
error_description:The specified ‘redirect_uri’ parameter is not valid for this client application.
VS has assigned a different port number to my resource server, but I’ve updated this everywhere in the code.
I somehow got around the invalid_request by downloading the solution again, setting the resource server port to 44308 in the project properties, as this is what’s in the source files. I ran those servers. I changed the client back so that redirect port would be 44308. Now when I log in the auth server redirects me to https://localhost:44308 where I get a 404. No Angular app pops up, nothing happens. When I try to manually navigate to the urls of the controllers, I get a 401. Nothing about this solution works.
Hi Peter, the github examples should work without changes, only the certs need to be excepted with the system running it. I run with IIS Express. I will check this again to make sure.
Greetings Damien
Hi Damien, sorry for the harsh words, I’m getting a bit frustrated here. Currently what happens when I log in is I get redirected to this url
https://localhost:44308/#resource=dataEventRecords&token_type=Bearer&access_token=eyJhbGciOiJSUzI1NiIsImtpZCI6IjlGRFcxUEpIS09VRUJNUEFBVTcxVDZPWkRaR1Q3Q0pBMFZBRE5VWloiLCJ0eXAiOiJKV1QifQ.eyJzdWIiOiI1MzQyM2IxMi0zNzI5LTRhOTktYjhhYy03OWY0NTBjOTMxOTAiLCJuYW1lIjoiamFAamEuY29tIiwicm9sZSI6WyJkYXRhRXZlbnRSZWNvcmRzLmFkbWluIiwiYWRtaW4iLCJkYXRhRXZlbnRSZWNvcmRzLnVzZXIiXSwidG9rZW5fdXNhZ2UiOiJhY2Nlc3NfdG9rZW4iLCJqdGkiOiIxNTcyYTc5YS05NzJkLTQ5OWItODZmOC1kYTI0ZWQ0NGRmNjMiLCJzY29wZSI6WyJvcGVuaWQiLCJkYXRhRXZlbnRSZWNvcmRzIl0sImF1ZCI6ImRhdGFFdmVudFJlY29yZHMiLCJhenAiOiJhbmd1bGFyNGNsaWVudCIsIm5iZiI6MTUyNTc2NTc5NSwiZXhwIjoxNTI1NzY5Mzk1LCJpYXQiOjE1MjU3NjU3OTUsImlzcyI6Imh0dHBzOi8vbG9jYWxob3N0OjQ0MzE5LyJ9.w9OiRlxZ-38EUKnmg0yIxClUG5WO5d2PMiRPaaAiQBi3ujUCfqNoQnJwaWeaG27TRbpOS9JWTVXhVqu-cqBWVvI802Ua9NdqNWzOvPGYZdxdGvoZdST7qHxZ4O5tEQ2tAgtSubel3Bei7lUy8_UN69Hq-VDMCCdh0dfTrzxvUIAzmYyQU3p0GiXs5bLT5Vc-2zuDp94lB9ZLIaup0_8B-bARyxQhjN92J1LsjbPZVnkMWgUbqFFZLIBNLY_5OHPxUyLtoGkkJFYvHOieX1RxhyQ8wnzIgAqdug675kKfcYI6IPZKLhALy7npr7XYwshdp33nBSFNZPSkNdbcuVZcPg&expires_in=3600&id_token=eyJhbGciOiJSUzI1NiIsImtpZCI6IjlGRFcxUEpIS09VRUJNUEFBVTcxVDZPWkRaR1Q3Q0pBMFZBRE5VWloiLCJ0eXAiOiJKV1QifQ.eyJzdWIiOiI1MzQyM2IxMi0zNzI5LTRhOTktYjhhYy03OWY0NTBjOTMxOTAiLCJuYW1lIjoiamFAamEuY29tIiwidG9rZW5fdXNhZ2UiOiJpZF90b2tlbiIsImp0aSI6IjVmNjgzMTIwLTQ5ZjEtNDQ1NC1iN2VhLTA1YTMzMTBiNGMyYiIsImF1ZCI6ImFuZ3VsYXI0Y2xpZW50Iiwibm9uY2UiOiJOMC4zNTQ0MTg5MTc5NjI0MjQ2NDE1MjU3NjU3ODQ3MTgiLCJhdF9oYXNoIjoiMzZWR3B2ZU9MbXpCSkVQUTByNUw0ZyIsImF6cCI6ImFuZ3VsYXI0Y2xpZW50IiwibmJmIjoxNTI1NzY1Nzk1LCJleHAiOjE1MjU3NjY5OTUsImlhdCI6MTUyNTc2NTc5NSwiaXNzIjoiaHR0cHM6Ly9sb2NhbGhvc3Q6NDQzMTkvIn0.bacTVNmv5cPOFujETe6nf0cfH-vEdCBtxI1QB8iZzjGBjXaKMTRhpbUvuq0yMFoSznTlKnZ2cc2KBT5TF8T_75EAJYAfb5Kh6j7SFWDPooXJNN_LqUC0d_X78kVV2TjCAaXUC7rgMvf1GB0WxKvBPaFYuFgjjPknBh2fniqbUaok6DnTsuE8h8WfX03NDXeSiy8uzP1hBvCuCwDwennoqVT-xMrywnOi1somBWuNhnCu1CdzMlvGEJWlRkmZ_e00voDR1gEl33wfayQFsCcFAL6ubrMn0MGLHeO8QPt_STdD3eoT5W91b6-gviEMQkNOgsiP31_l5qg0EpSS7-IGTw&state=15257657847180.41978672363962644
and there I get a 404. I know the resource server is running, because this url https://localhost:44308/index.html returns the index page.
So the login to the STS is finished. The return URL is not been handled by the client application, maybe server routing first. You need that the client routing handles the return URL. Disable the server routing for this return URL. Note it works when you open the index static page because this is not handled by the server routing.
Greetings Damien
Thanks, I’ll try that. Did you get a chance to check your sample?
I have updated the example, and tested it. seems to work
Thanks. I’ve been sitting here for the past 3 hours just trying to get the projects to run. First I had to update my VS, as apparently it was old. Then I had to update then .NET SDK, then the .NET Core SDK. Then the ASPNET core app failed while starting, the ASPNET core web server output said it was unable to locate the correct aspnet core app version. It asked for 2.1.0-rc1, so I installed that one specifically. Now I get a compile time error:
Error The project was restored using Microsoft.NETCore.App version 2.1.0-preview2-26406-04, but with current settings, version 2.1.0-rc1 would be used instead. To resolve this issue, make sure the same settings are used for restore and for subsequent operations such as build or publish. Typically this issue can occur if the RuntimeIdentifier property is set during build or publish but not during restore.
Could you possibly tell me what to install/uninstall or which setting to change? Cause I’m at the end of my wits and just about ready to take out a hammer and smash my machine to bits.
Nope, after spending several hours trying to get this updated version to run, I got to the exact same situation I had last time: auth server running, resource server running, client running. I am able to register a user, log in, and then am redirected to the resource server where I get a 404.
I think it’s time for me to declare defeat and stop spending my time on this sample. I was really happy when I first saw it, cause I thought I could get my little side project started, without having to go through the pain of having to code the authentication infrastructure myself. But it keeps not working no matter what I do and I’m at the end of my mental capacity to continue trying.
So long and thanks for all the help.
Hi Peter
Sorry to hear that 😦 Hope you have success building the new solution.
Greetings Damien
[…] Implementing OpenID Implicit Flow using OpenIddict and Angular by Damien Bowden. […]
Hi Damien,
I created a solution based on your code. I’m not using Angular but instead added a test Console app and also added ClientCredentialsFlow based off the original Aridka sample. My resource server project is an empty project with the default WeatherForecast stuff.
I do get a token, however, I get 401 Unauthorized when I access the api.
Any ideas what I could be doing wrong?
Hi Ivan-Mark
The access token you send to the API has different claims to the ones expected in the API. Open the access token using jwt.io or jwt.ms and check the claims against the ones validated in the API. You might need to turn of the decyption in the token to check like this. Openiddict has a seetings for this, I think.
Or you could add debugging, logging to the API with PII and check the message why the token was rejected.
Greetings Damien
Hi Damien,
I want to use Openiddict with a react native app(client) authentication API(outside protected resource to make sure the user is valid) and a resource API to pull data from endpoints.
They need to be seperate from the Openiddict project. Is my best bet to register them all as clients to that Openiddict server and give scope permissions to each one? What about introspection? Is that applicable in my situation?
Thanks
Cody.
[…] Implementing OpenID Code Flow with PKCE using OpenIddict and Angular […]
Hello all, I need angular 13 and asp.net core web api 6 version can any one has link give me. thanks
Just check this tag:
https://github.com/damienbod/AspNetCoreOpeniddict/releases/tag/net6
Greetings Damien
Is it possible to expand the article and code on how the Openiddict authserver handles logout and revocation.